新建项目 类
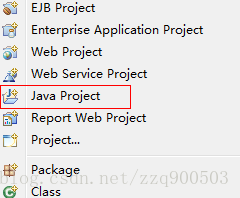
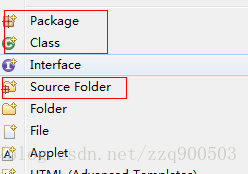
得到结构如下:

TestIo类中的代码:
package Test;
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.InputStreamReader;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Calendar;
public class TestIo {
private static final DateFormat DATE_FORMAT = new SimpleDateFormat(
"yyyy-MM-dd");
public static void writeToFile(String fileName, String content) {
String time = DATE_FORMAT.format(Calendar.getInstance().getTime());
File dirFile = null;
try {
dirFile = new File("e:\\" + time);
if (!(dirFile.exists()) && !(dirFile.isDirectory())) {
boolean creadok = dirFile.mkdirs();
if (creadok) {
System.out.println(" ok:创建文件夹成功! ");
} else {
System.out.println(" err:创建文件夹失败! ");
}
}
} catch (Exception e) {
e.printStackTrace();
}
String fullPath = dirFile + "/" + fileName + ".txt";
write(fullPath, content);
}
/**
* 写文件
*
* @param path
* @param content
*/
public static boolean write(String path, String content) {
String s = new String();
String s1 = new String();
BufferedWriter output = null;
try {
File f = new File(path);
if (f.exists()) {
} else {
System.out.println("文件不存在,正在创建...");
if (f.createNewFile()) {
System.out.println("文件创建成功!");
} else {
System.out.println("文件创建失败!");
}
}
BufferedReader input = new BufferedReader(new FileReader(f));
while ((s = input.readLine()) != null) {
s1 += s + "\n";
}
System.out.println("原文件内容:" + s1);
input.close();
s1 += content;
output = new BufferedWriter(new FileWriter(f));
output.write(s1);
output.flush();
return true;
} catch (Exception e) {
e.printStackTrace();
return false;
} finally {
if (output != null) {
try {
output.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
public static String readFile(String str) {
File file = new File(str);
String line;
InputStreamReader isr= null;
try {
isr = new InputStreamReader(new FileInputStream(file), "utf-8");
BufferedReader in = new BufferedReader(isr);// 包装文件输入流,可整行读取
StringBuilder sb = new StringBuilder();
while ((line = in.readLine()) != null) {
sb.append(line);
}
return sb.toString();
} catch (FileNotFoundException e) {
e.printStackTrace();
return null;
}// 创建文件输入流
catch (IOException e) {
e.printStackTrace();
return null;
} finally {
if (isr != null) {
try {
isr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
public static void main(String[] args) {
String fileName ="test";
String content="Tonight,we are young";
writeToFile(fileName, content);
// String ReadString= readFile("e:/2013-09-11/test.txt");
// System.out.print(ReadString);
}
} |